Python Tips and Tricks
Python is one of the most loved programming languages. Wanna know why it is so popular? Here I’ll show you 7 python tips and tricks used by many developers when writing code. My name is Husnain and I’m a Software Engineer who smokes Python everyday.
#1
Want to swap two numbers?
Here how you will do In any programming language
var a = 10;
var b = 20;
var tmp = a;
a = b;
b = tmp ;
This is how you do this in python
a = 10
b = 20
a,b = b,a
Cleaaaaannnn Right?
#2
Let’s say you want to write a sentence n number of times
This is how you do in other programming languages
for(var i = 0; i<n; i++)
print(‘post4everyone’);
This is how you do this in python
print(‘post4everyone’ * n)
#3
If you want to check if the element is in a list or not
This is how you do in other programming languages
function validate(collection, x){
for (var el in collection){
if (el == x)
return true
return false
}
}
This is how you do this in python
def validate(collection, x)
return x in collection
Lit right?
#4
Lets say you want to assign first and last members of a list to other variables and the middle part to another
This is how you do in other languages
lst = ["A", "B", "C", "D", "E"]
a = lst[0]
c = lst[lst.length - 1]
b = Array()
for(var i = 1; i < lst.size - 1; i++)
b += lst[i]
This is how you do this in python.
lst = ["A", "B", "C", "D", "E"]
a, *b, c = lst
Now this is beauty
#5
Do you want to reverse a list?
This is how you do in other programming languages
function reversList(original){
var rev = new Array;
for(var i = original.length-1; i >= 0; i--) {
rev.push(input[i]);
}
return rev;
}
This is how you do this in python
def reversList(original):
return reverse(original)
Yes python has a built in reverse method.
Wanna know another way to reverse list?
def reversList(original):
return original[::-1]
Didn’t understand this? See the next point
#6
Python comes with a pretty cool feature which no other programming language has. One of them is list slicing.
Let’s say you want to slice a list from 2nd element to 5th this is how you do in other programming languages
var lst = [1,2,3,4,5,6,7,8,9];
x = Array();
for(var i = 2; i < 6; i++)
x += lst[i];
This is how you do this in python
lst = [1,2,3,4,5,6,7,8,9]
x = lst[2:6]
So we are asking python to assign the members between 2nd and 6th index (the last index is not inclusive)
#7
List comprehensions is the single most powerful and most loved feature of python. I wish every programming language have this one for sure.
Let’s say you want to filter out only even numbers from a list, this is how you do in other programming languages
function getEven(numbers){
even = Array();
for(var num in numbers){
if (num % 2 == 0)
Even += num;
}
return even;
}
This is how you do in python
def getEven(numbers):
return [num for num in numbers if num %2 == 0]
Posted: 19 Dec 2020
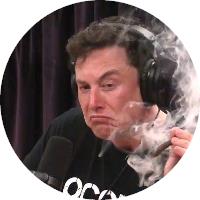
Visit for more
() () ()

Hussnain
I thought you only smoke weed, python too?
()
()
(1509 days, 42 minutes ago)
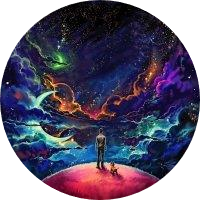
Sana
Smoking post in fogy days :P
()
()
(1509 days, 27 minutes ago)
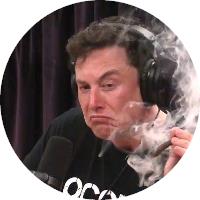
HusnainAbbas
You might surprise if I tell you what else I smoke. *wink wink*
()
()
(1505 days, 9 hours, 13 minutes ago)